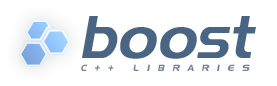
|
Boost Users :
|
Yes, I have often wondered why those were left out. Several
comments:
1) Sometimes I use Boost::Tuple instead of std::pair just because it has
getters and that makes for_each useable.
2) This would be an excellent point to make when discussing why getters
are good programming.
3) I wrote my own templates to do this, they're very simple.
template
<
typename
P >
typename
P::first_type
const
& const_first_of_pair(P
const
& p) {
return
p.first; }
template
<
typename
P >
typename
P::first_type& first_of_pair(P& p) {
return
p.first; }
template
<
typename
P >
typename
P::second_type
const
& const_second_of_pair(P
const
& p) {
return
p.second; }
template
<
typename
P >
typename
P::second_type& second_of_pair(P& p)
{
return
p.second; }
The const/non-const versions turn out to be necessary in practice.
While you want the const versions for this particular application, there
are other times when the std::pair is mutable and having a non-const
accessor is required.
Use looks like this, for a map where I want to do an internal data update
on each object in an STL container (i.e., call a non-const method on each
value type in the container).
std::for_each(m_lines.begin(), m_lines.end(), bind(&Line::split,
bind(&second_of_pair<container::value_type>, _1)));
Implementation issues:
1) I made them functions instead of functors because I always pass them
to Boost.Bind and functors add weight without benefit in that case.
2) You have to pass in the pair type, not the STL container type, because
I didn't have the energy to write the MPL logic to detect the
"container-ness" of the template argument. I think that could
be done, but I didn't think about it very much.
3) Could Boost.get be extended so that you could use
"get<1,container::value_type>" instead of
"second_of_pair<container::value_type>"?
At 05:04 AM 7/24/2006, gast128 wrote:
Dear all,
in our company we use the STL map frequently. However one often wants to
iterate over the key_type only (or mapped type, or make desicions for the
key
type, and take action on its corresponded map type).
A select1st or select2nd would already a great help.
Boost-users list run by williamkempf at hotmail.com, kalb at libertysoft.com, bjorn.karlsson at readsoft.com, gregod at cs.rpi.edu, wekempf at cox.net